In this topic you will learn how to programmatically navigate to a new URL when a video has completed playing.
Getting started with the basic code
Before you see how to implement the code to navigate to a new URL when a video has completed playing, first examine the starting code. The HTML page contains required elements plus:
- The Brightcove player code.
- JavaScript needed to enable programmatic control of the player.
<!-- Start of Brightcove Player -->
<div style="display:none">
</div>
<!--
By use of this code snippet, I agree to the Brightcove Publisher T and C
found at https://accounts.brightcove.com/en/terms-and-conditions/.
-->
<script type="text/JavaScript" src="http://admin.brightcove.com/js/BrightcoveExperiences.js"></script>
<object id="myExperience1871207962001" class="BrightcoveExperience">
<param name="bgcolor" value="#FFFFFF" />
<param name="width" value="480" />
<param name="height" value="270" />
<param name="playerID" value="1811612799001" />
<param name="playerKey" value="AQ~~,AAAAAFigZAk~,3mLHk34QtPe2oGNsUdHkE6E75zTfMG58" />
<param name="isVid" value="true" />
<param name="isUI" value="true" />
<param name="dynamicStreaming" value="true" />
<param name="@videoPlayer" value="1871207962001" />
<!-- smart player api params -->
<param name="includeAPI" value="true" />
<param name="templateLoadHandler" value="onTemplateLoad" />
<param name="templateReadyHandler" value="onTemplateReady" />
</object>
<!--
This script tag will cause the Brightcove Players defined above it to be created as soon
as the line is read by the browser. If you wish to have the player instantiated only after
the rest of the HTML is processed and the page load is complete, remove the line.
-->
<script type="text/JavaScript">brightcove.createExperiences();</script>
<!-- End of Brightcove Player -->
<script type="text/JavaScript">
var player,
APIModules,
videoPlayer;
function onTemplateLoad(experienceID){
player = brightcove.api.getExperience(experienceID);
APIModules = brightcove.api.modules.APIModules;
}
function onTemplateReady(evt){
videoPlayer = player.getModule(APIModules.VIDEO_PLAYER);
videoPlayer.play();
}
</script>
This code is explained in the Smart Player API Samples: Basic Setup document.
Implementing navigation redirect on video complete
The implementation of this functionality consists of three main parts:
- Add an event listener for video completion.
- Determine a URL to which navigation should proceed. In this example you will see how to use the
linkURL
property which is associated with the video playing. The new destination's URL could also be hard-coded or read from a custom field associated with the video. - Use the
window.location
object'shref
property to navigate to the intended page.
Adding an event listener
In the onTemplateReady()
event handler add and event listener to the player. The event to listen for is brightcove.api.events.MediaEvent.COMPLETE
and reference an event handler function, in this case named myCompleteHandler
.
videoPlayer.addEventListener( brightcove.api.events.MediaEvent.COMPLETE, myCompleteHandler);
Retrieving the URL
In this example the URL to which to navigate will be read from the video information that is stored in the Related Link section in the Basic tab of the video properties. This information is in a property named linkURL
in the video object.
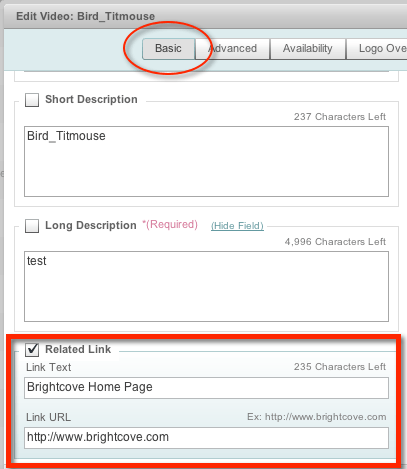
To retrieve this value call the getCurrentVideo()
function in the onTemplateReady
event handler. Use an anonymous function that accepts a parameter, then retrieve from the video data transfer object (DTO) the linkURL
value and store it in a variable.
videoPlayer.getCurrentVideo( function (videoDTO) {
redirectURL = videoDTO.linkURL;
});
Navigating to the URL
Finally, in the event handler defined earlier in the addEventListener()
method, use the window.locatio
n object's href
property to redirect the browser to the URL.
function myCompleteHandler(){
window.location.href = redirectURL;
}
Final script block
The following code shows the implementation of the three parts:
<script type="text/javascript">
var player,
APIModules,
videoPlayer,
redirectURL;
function onTemplateLoad(experienceID){
player = brightcove.api.getExperience(experienceID);
APIModules = brightcove.api.modules.APIModules;
}
function myCompleteHandler(){
window.location.href = redirectURL;
}
function onTemplateReady(evt){
videoPlayer = player.getModule(APIModules.VIDEO_PLAYER);
videoPlayer.play();
videoPlayer.addEventListener( brightcove.api.events.MediaEvent.COMPLETE, myCompleteHandler);
videoPlayer.getCurrentVideo( function (videoDTO) {
redirectURL = videoDTO.linkURL;
});
}
</script>
To actually see this page in action, and view the complete source code, it is located here.
Related Topics
- Smart Player API Samples: Basic Setup
- Information on the addEventListener() method