In this topic you will learn how to determine when a rendition changes and get details of the rendition currently playing. Since there is not rendition change event to rely upon, the solution approach is to periodically retrieve rendition information and check to see if it is different from the last rendition playing. Check out the completed page here, and, of course, use View Source to see the complete source code.
Preparing to use the Smart Player API
You must do a few simple steps to use the Smart Player API in an HTML page. You will start with the basic Player code embedded in an HTML document. The Player code can be copied from Video Cloud Studio. Here is an example of the Player code:
<!-- Start of Brightcove Player -->
<div style="display:none">
Used for related videos API sample
</div>
<!--
By use of this code snippet, I agree to the Brightcove Publisher T and C
found at https://accounts.brightcove.com/en/terms-and-conditions/.
-->
<script language="JavaScript" type="text/javascript" src="http://admin.brightcove.com/js/BrightcoveExperiences.js"></script>
<object id="myExperience922656010001" class="BrightcoveExperience">
<param name="bgcolor" value="#FFFFFF" />
<param name="width" value="480" />
<param name="height" value="270" />
<param name="playerID" value="2344262015001" />
<param name="playerKey" value="AQ~~,AAAA1oy1bvE~,ALl2ezBj3WHB4SZjVHPI3HSdWBlOCXX4" />
<param name="isVid" value="true" />
<param name="isUI" value="true" />
<param name="dynamicStreaming" value="true" />
<param name="@videoPlayer" value="922656010001" />
</object>
<!--
This script tag will cause the Brightcove Players defined above it to be created as soon
as the line is read by the browser. If you wish to have the player instantiated only after
the rest of the HTML is processed and the page load is complete, remove the line.
-->
<script type="text/javascript">brightcove.createExperiences();</script>
<!-- End of Brightcove Player -->
Next add the three statements just above the closing </object>
tag to enable the player to use the Smart Player API.
<!-- smart player api params -->
<param name="includeAPI" value="true" />
<param name="templateLoadHandler" value="onTemplateLoad" />
<param name="templateReadyHandler" value="onTemplateReady" />
For more information about getting started with the Smart Player API see Get Started with the Smart Player API.
Understanding the logic of the code
Here is a high-level look at the logic of retrieving the rendition data
- In the
onTemplateReady
event handler get theVIDEO_PLAYER
module and play the video - Use the
getCurrentRendition()
method to retrieve the current rendition information and use an anonymous function for the event handler - In the event handler perform the following tasks
- Display the current rendition information
- Assign the rendition encoding rate to a variable (this value will be used to see if a rendition change has occurred)
- Add an event listener on the
PROGRESS
event
- In the
PROGRESS
event handler perform the following tasks- Check if the video has completed
- Get the current rendition information
- Check to see if the encoding rate has changed from the last check
- If the encoding rate has changed, perform the following tasks
- Display the new rendition information
- Assign the new rendition's encoding rate to a variable (this value will be used to see if a rendition change has occurred)
The results of this code will look something like this:
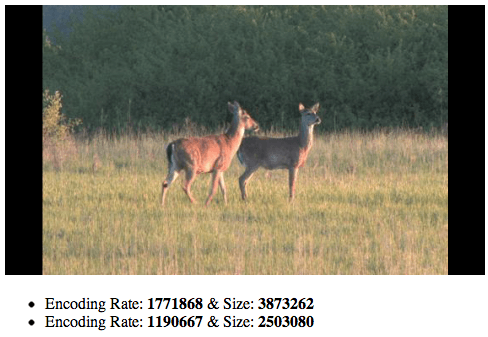
Retrieving rendition information
The key method to get rendition information is, not surprisingly, getCurrentRendition()
. This method returns the data transfer object (DTO) for the current rendition playing. The contents of the DTO can be learned two ways, via the documentation and logging the object in a browser's developer tools. The two screenshots show those two options.
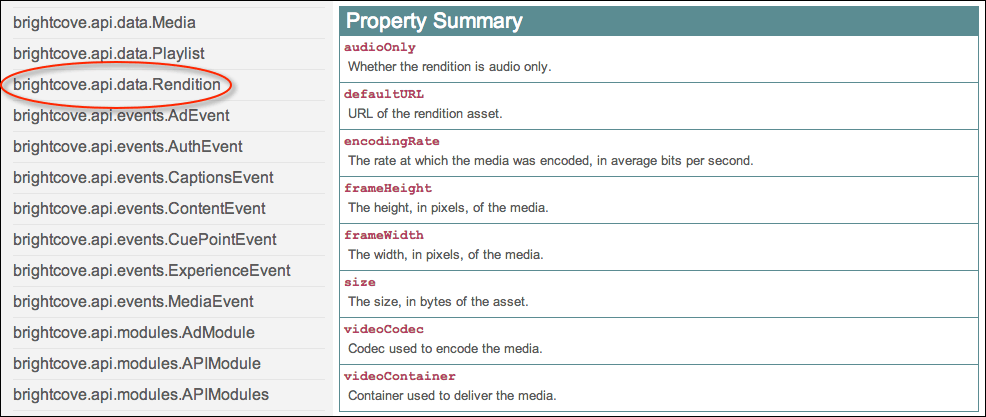
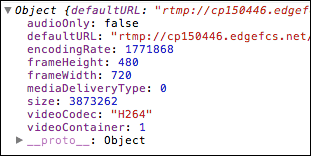
The following code is a fragment from the onTemplateReady
event handler that gets the current rendition information then displays it and assigns the encodingRate
property of the DTO to a variable.
videoPlayer.getCurrentRendition( function(renditionDTO){
displayRendition(renditionDTO);
currentEncodingRate = renditionDTO.encodingRate;
});
Creating the PROGRESS event handler
The progress handler needs to check to see if the rendition changes. The handler is called every fraction of a second by the PROGRESS event. The first if
statement checks to be sure the video is still playing (checking if the total length [duration
] of the video is very close to the current playing time [position
]). If the video is still playing, the code then gets the current rendition playing. The second if
statement checks to see if the current encoding rate is different from the old encoding rate. If it is different, the rendition has changed and the new rendition information is displayed. The variable holding the current encoding rate is also updated for further comparison. The code for the event handler appears as follows.
var onProgress = function(evt){
if ((evt.duration - evt.position) > .1) {
videoPlayer.getCurrentRendition( function(renditionDTO){
if (currentEncodingRate != renditionDTO.encodingRate){
displayRendition(renditionDTO);
currentEncodingRate = renditionDTO.encodingRate;
}
});
}
};
Displaying the rendition information
Displaying the rendition information is straight forward and uses no features of the Smart Player API. An HTML <div>
is created, then the JavaScript innerHTML
property is used to add list items to the HTML. The function to display the rendition information follows.
var displayRendition = function (renditionDTO){
var renditionString = '<li>Encoding Rate: <strong>' + renditionDTO.encodingRate + '</strong> & Size: <strong>' + renditionDTO.size + '</strong>';
renditionDisplay.innerHTML += renditionString;
}