In this topic you will learn how to display video information using the Smart Player API. Check out the completed page here, and, of course, use View Source to see the complete source code.
Preparing to use the Smart Player API
You must do a few simple steps to use the Smart Player API in an HTML page. You will start with the basic Player code embedded in an HTML document. The Player code can be copied from Video Cloud Studio. Here is an example of the Player code:
<!-- Start of Brightcove Player -->
<div style="display:none">
Used for related videos API sample
</div>
<!--
By use of this code snippet, I agree to the Brightcove Publisher T and C
found at https://accounts.brightcove.com/en/terms-and-conditions/.
-->
<script language="JavaScript" type="text/javascript" src="http://admin.brightcove.com/js/BrightcoveExperiences.js"></script>
<object id="myExperience922656010001" class="BrightcoveExperience">
<param name="bgcolor" value="#FFFFFF" />
<param name="width" value="480" />
<param name="height" value="270" />
<param name="playerID" value="2344262015001" />
<param name="playerKey" value="AQ~~,AAAA1oy1bvE~,ALl2ezBj3WHB4SZjVHPI3HSdWBlOCXX4" />
<param name="isVid" value="true" />
<param name="isUI" value="true" />
<param name="dynamicStreaming" value="true" />
<param name="@videoPlayer" value="922656010001" />
</object>
<!--
This script tag will cause the Brightcove Players defined above it to be created as soon
as the line is read by the browser. If you wish to have the player instantiated only after
the rest of the HTML is processed and the page load is complete, remove the line.
-->
<script type="text/javascript">brightcove.createExperiences();</script>
<!-- End of Brightcove Player -->
Next add the three statements just above the closing </object>
tag to enable the player to use the Smart Player API.
<!-- smart player api params -->
<param name="includeAPI" value="true" />
<param name="templateLoadHandler" value="onTemplateLoad" />
<param name="templateReadyHandler" value="onTemplateReady" />
For more information about getting started with the Smart Player API see Get Started with the Smart Player API.
Understanding the logic of the code
Here is a high-level look at the logic for displaying video information
- In the
onTemplateReady
event handler get theVIDEO_PLAYER
module and play the video - Use the
getCurrentVideo()
method to retrieve the current video information and use an anonymous function for the event handler - Display the video title and short description
- Use a
for
loop to iterate over thetags
array information and display them - Use a
for-in
loop to iterate over thecustomFields
object info and display them - Use a method for improved duration display
The results of this code will look something like this:
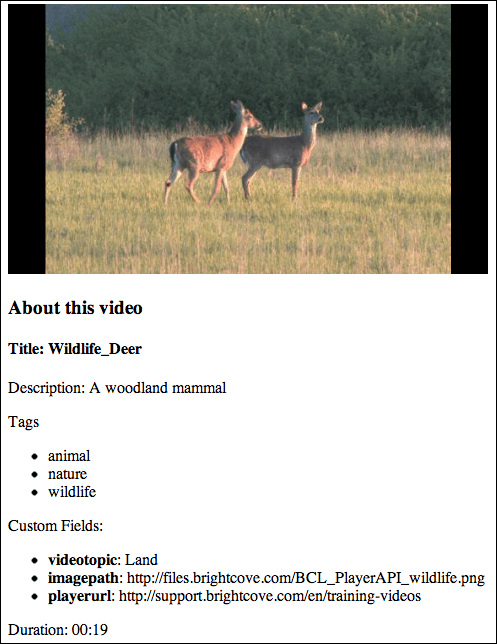
Retrieving video information
The key method to get video information is, not surprisingly, getCurrentVideo()
. This method returns a data transfer object (DTO) containing key information about the currently video playing. The contents of the DTO can be discovered two ways, via the documentation and logging the object in a browser's developer tools. The two screenshots show those two options. (Note the API documentation is only a partial listing of the properties.)
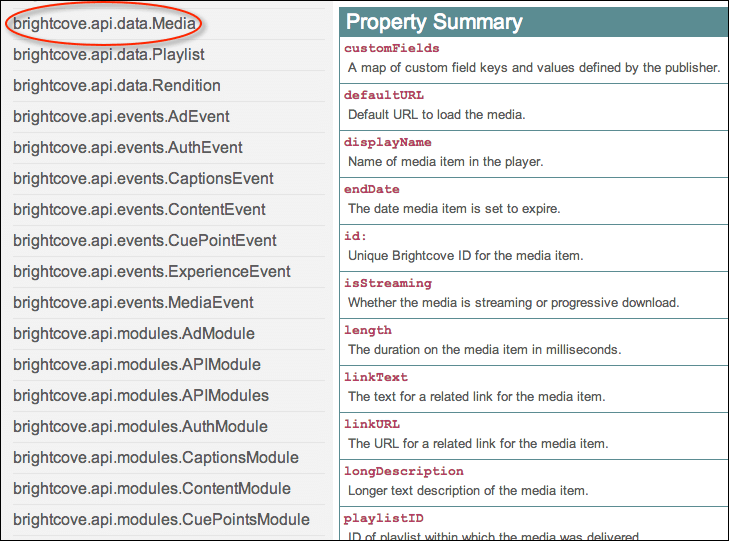
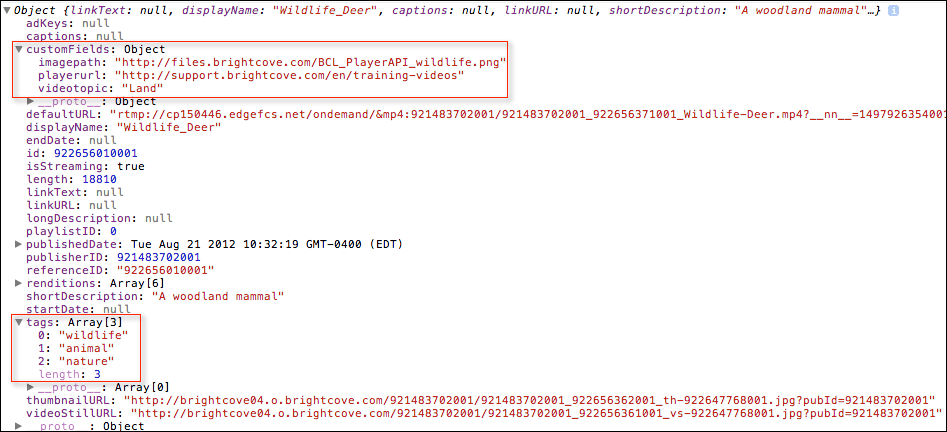
Note the custom field information is contained in an object and the tag information is contained in an array. When displaying this information using JavaScript you will need to loop over these data structures.
You use the getCurrentVideo()
method and need an event handler to display the returned information. In this case an anonymous function is used, which simply means you define the event handler in the parentheses of the method call. This means the method will be called, and when data is returned the function defined will be executed, accepting a parameter, in this case using the logical name videoDTO
.
videoPlayer.getCurrentVideo( function(videoDTO){
[event handler definition here]
});
In the event handler, displaying data that is NOT stored in a complex data structure is straight forward. You simply use the object.property name from the DTO, for instance, videoDTO.displayName
and videoDTO.shortDescription
.
The technique used to display information in this example will be to build a long string of HTML, then use the JavaScript innerHTML
property to place the HTML into the page. This is a simple, but not a best practice, approach to build the display. A better approach would be to use a JavaScript templating system like Handlebars or EJS, but it is not used here so as not to cloud the Smart Player API content.
Displaying video tags
To display the video tags, which are stored in an array, you can loop over the tags
property of the DTO that contains that array. In this example, HTML list items are built and added to the HTML string.
for (var i = 0, max = videoDTO.tags.length; i < max; i++) {
infoForDisplay += "<li>" + videoDTO.tags[i] + "</li>";
}
Displaying custom fields
You can view the custom fields assigned to a video in Video Cloud Studio. For the video above you see three custom fields have been assigned values.
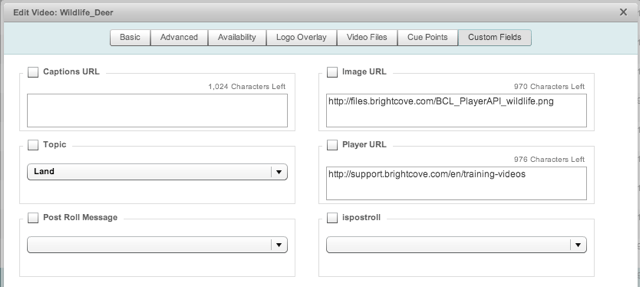
This data is sent in the DTO as an object and can be represented as shown in the following table.
key | value |
---|---|
videotopic | Land |
imagepath | http://files.brightcove.com/... |
playerurl | http://support.brightcove.com/training ... |
Displaying these custom fields is much like displaying tags, as both are stored in a complex data structure. The difference lies in the fact that custom fields are stored in an object, hence having both a value and a key associated with that value, as shown in the table above. A for-in loop works well for displaying data from an object, as shown in the following code.
infoForDisplay += "<p>Custom Fields:</p><ul>";
for (var key in videoDTO.customFields) {
infoForDisplay += "<li><strong>" + key + "</strong>: " + videoDTO.customFields[key] + "</li>";
}
infoForDisplay += "</ul>";
Displaying the duration
To display the duration you can use the getVideoDuration()
method. Although the length
property is available via the videoDTO, this method will format the date in a better format than the millisecond value of length
. The method takes two parameters, the first a boolean whether to format the result or not, and the second is the callback event handler. In the code below the duration will be formatted, and the callback event handler is defined as an anonymous function.
var displayDuration = function(){
videoPlayer.getVideoDuration( true, function(duration) {
displayInfo.innerHTML += "<p>Duration: " + duration + "</p>";
});
};