This document outlines best practices for embedding Video Cloud media such as images, videos, and specific renditions in web or mobile apps.
Accessing Video Cloud media via URLs
There may be cases where you want to access media files in your Video Cloud account directly, rather than through data-binding in a player. You might be displaying information about your videos in a page of a web app, or accessing specific media files in a mobile app to meet the requirements of an app store.
The Video Cloud Media API provides an easy way to access media data, including metadata and URLs for assets. When you use the Media API to access media assets, it is important to do it in the right way, so that links in your app will not break at some point.
URLs will change!
The most important thing to remember is that the URLs for images, video files, and renditions are not fixed. Brightcove reconfigures the storage of media assets from time to time to meet requirements of some change in our internal systems, to improve performance, or for other reasons. When this happens, URLs for specific assets will change, so if your app is relying on hard-coded URLs to theses assets, the links will break at some point.
Preventing broken links
The best way to prevent links to media from breaking is to retrieve them from Video Cloud at runtime using the Media API. Media API calls can be made on the server or client side, and there are wrappers for various programming languages on the Brightcove Open Source Project site to simplify making these calls. Below is a simple example that uses the JavaScript Media API wrapper to retrieve data for a set of videos and insert it into the HTML:
<html><head><title>Media API Sample</title><meta http-equiv="Content-Type" content="text/html; charset=utf-8" /><!-- Javascript Media API wrapper from http://docs.brightcove.com/en/video-cloud/open-source/index.html --><script type="text/javascript" src="http://files.brightcove.com/bc-mapi.js"></script></head><body><h1>Media API Sample</h1><!-- Start of Brightcove Player --><script language="JavaScript" type="text/javascript" src="http://admin.brightcove.com/js/BrightcoveExperiences.js"></script><object id="myExperience" class="BrightcoveExperience"><param name="bgcolor" value="#FFFFFF" /><param name="width" value="480" /><param name="height" value="270" /><param name="playerID" value="921267190001" /><param name="playerKey" value="AQ~~,AAAA1oy1bvE~,ALl2ezBj3WG3MLvDx9F9zkV06cNK00ey" /><param name="isVid" value="true" /><param name="isUI" value="true" /><param name="dynamicStreaming" value="true" /><!-- params for Universal Player API --><param name="includeAPI" value="true" /><param name="templateReadyHandler" value="BCL.onTemplateReady" /></object><script type="text/javascript">brightcove.createExperiences();</script><!-- End of Brightcove Player --><fieldset><legend>Videos</legend><div id="results"></div></fieldset><br><!-- This is the script to modify for the exercise --><script type="text/javascript"> // BCL Media API search maker -- adapted from JS-MAPI on http://docs.brightcove.com/en/video-cloud/open-source/index.html // namespace to keep all the "global" vars together var BCL = {}; // placeholder - params for API call BCL.params = {}; // Media API read token BCMAPI.token = "WDGO_XdKqXVJRVGtrNuGLxCYDNoR-SvA5yUqX2eE6KjgefOxRzQilw.."; // set the callback for Media API calls BCMAPI.callback = "BCL.onSearchResponse"; // set the filter BCL.params.any = "tag:nature"; BCMAPI.search(BCL.params); BCL.onSearchResponse = function(jsonData) { var str = ""; for (var index in jsonData.items) { str += "<a onclick=\"BCL.playVideo(" + jsonData.items[index].id + ")\" style=\"cursor:pointer\"><img src=\"" + jsonData.items[index].thumbnailURL + "\"/><br/><small>" + jsonData.items[index].name + "</<small></a><hr/>"; } document.getElementById("results").innerHTML = str; } // Player API scripting // event listener for the player being ready BCL.onTemplateReady = function (event) { BCL.player = brightcove.api.getExperience("myExperience"); // get a reference to the video player BCL.videoPlayer = BCL.player.getModule(brightcove.api.modules.APIModules.VIDEO_PLAYER); } // play video function BCL.playVideo = function(videoID) { BCL.videoPlayer.loadVideoByID(videoID); }</script></body></html>
Rate limiting
The Media API is intended to be used as a tool for integrating your CMS or other backend systems with Video Cloud. It is not built for high-volume runtime access.
Read methods
Access frequency for read requests should be limited to 5 requests per second, and for long-running requests (returning large datasets), no more than 10 concurrent requests are permitted.
Write methods
Write requests are single-threaded, so you should wait for a response to a request before submitting another one.
Caching Media API call results
For page performance or other reasons, you may not want to make the Media API calls to retrieve data on each page load. In that case, you can create a caching layer to hold the results locally. Setting up caching can be done in a variety of ways, but the basic logic is:
- Make the Media API call(s) to retrieve the data
- Cache the data on your local caching server
- Pull URLs and other data from your caching server
- Refresh the cache by repeating the Media API call(s) at least every six hours
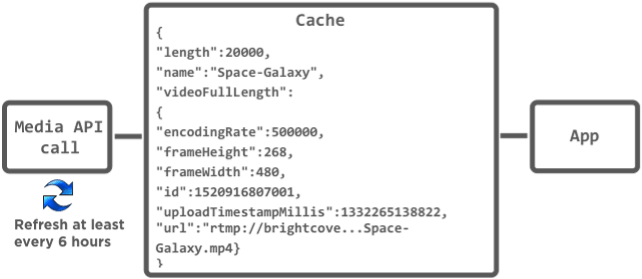